Managing Python environments is a critical skill for developers, ensuring clean, reproducible, and isolated development setups. A well-managed Python environment is the secret sauce of successful projects. It ensures everyone's cooking with the same ingredients.
This comprehensive guide covers a wide range of options for managing Python environments, detailing their pros, cons, and usage instructions. We'll explore various use cases and include insights from experienced developers to help you make informed decisions.
Python's flexibility and vast ecosystem of libraries make it a powerful tool for various applications, from web development to data science. However, this versatility can lead to challenges when managing different projects with conflicting dependencies or Python versions. Effective environment management solves these issues by:
Isolating project dependencies
Ensuring reproducibility across different machines
Allowing experimentation without affecting system-wide installations
Facilitating collaboration by standardizing development environments
As one experienced developer on Reddit put it:
Managing Python environments is like having separate kitchens for each cuisine you cook. It keeps flavors from mixing where they shouldn't and ensures you always have the right ingredients on hand.
Let's dive into the most popular options for managing Python environments, along with their strengths, weaknesses, and practical applications.
Quick comparison table
This table provides a comprehensive comparison of nine popular Python environment management tools, evaluating them across key features such as ease of use, platform compatibility, and dependency handling capabilities. Each tool is rated on a five-star scale for various attributes, offering a quick visual guide to their strengths and limitations.
Whether you're a beginner looking for a straightforward solution like venv, or an advanced user seeking a robust, all-encompassing platform like Docker, this overview covers the essential aspects to inform your choice.
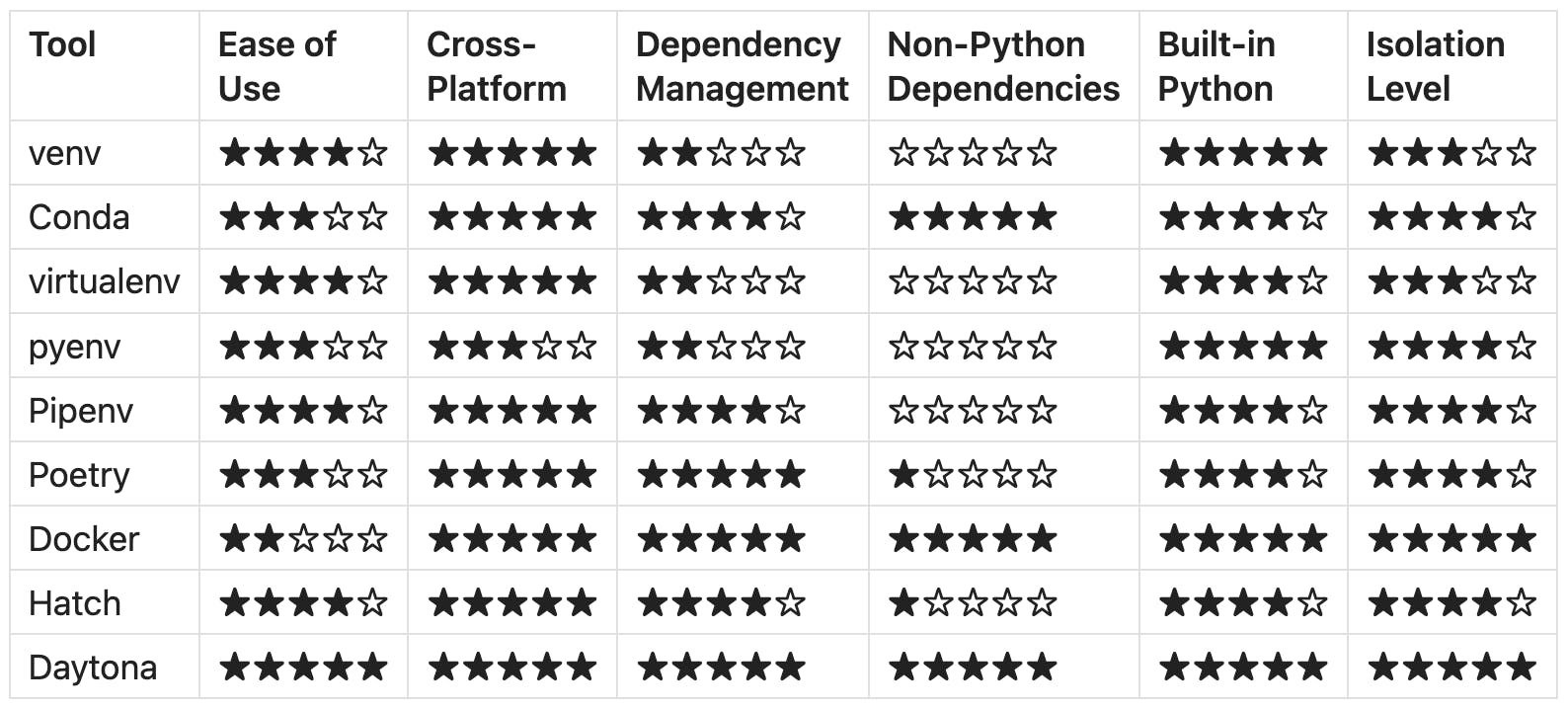
Jake VanderPlas, author of "Python Data Science Handbook" and core contributor to several scientific Python libraries, emphasizes the importance of reproducibility in data science workflows:
In data science, reproducibility is key. Tools like Conda have been game-changers, allowing us to create environments that capture not just Python packages, but also system-level libraries and even specific compiler versions. This level of control is crucial when working with complex numerical libraries or machine learning frameworks.
1. Venv (Virtual Environments)
Venv is like the Swiss Army knife of Python environment management - it's built-in, does the job well, and is always there when you need it.
Overview:
Built-in module for Python 3.3+
Creates isolated Python environments for specific projects
Pros:
Lightweight and fast
Integrated with Python; no extra installations needed
Simple to set up and use
Cons:
Limited to Python dependencies
Manual dependency management
Usage:
1# Create environment2python -m venv myenv34# Activate environment (Windows)5myenv\Scripts\activate67# Activate environment (macOS/Linux)8source myenv/bin/activate910# Install packages11pip install package_name1213# Create requirements.txt14pip freeze > requirements.txt1516# Install from requirements.txt17pip install -r requirements.txt1819# Deactivate environment20deactivate
2. Conda
Conda is the Swiss Army knife that comes with its own toolbox. It's powerful, but you need to learn how to use all those tools effectively.
Overview:
Package and environment management system for multiple languages
Part of Anaconda distribution, also available via Miniconda
Pros:
Manages Python and non-Python dependencies
Strong community support, especially in data science
Cross-platform compatibility
Cons:
Steeper learning curve
Heavier and slower setup compared to venv
Usage:
1# Install Conda (skip if already installed)2# Download and run Miniconda installer from https://docs.conda.io/en/latest/miniconda.html34# Create environment5conda create --name myenv python=3.867# Activate environment8conda activate myenv910# Install packages11conda install numpy pandas matplotlib1213# Create environment file14conda env export > environment.yml1516# Create environment from file17conda env create -f environment.yml1819# Deactivate environment20conda deactivate
3. Virtualenv
Overview:
Similar to venv, works with Python 2 and 3
Pros:
Compatible with both Python 2 and 3
More customization options than venv
Cons:
Requires separate installation
Largely redundant for Python 3.3+ users
Usage:
1# Install virtualenv2pip install virtualenv34# Create environment5virtualenv myenv --python=python3.867# Activate environment (Windows)8myenv\Scripts\activate910# Activate environment (macOS/Linux)11source myenv/bin/activate1213# Install packages14pip install package_name1516# Create requirements.txt17pip freeze > requirements.txt1819# Deactivate environment20deactivate
4. Pyenv + Virtualenv
Pyenv is like having a time machine for Python. You can jump between versions effortlessly, making it a lifesaver for maintaining legacy code.
Overview:
Pyenv manages multiple Python versions
Pyenv-virtualenv plugin manages virtual environments
Pros:
Easy switching between Python versions
Combines benefits of pyenv and virtualenv
Cons:
More complex setup
Requires installation of plugins
Usage:
1# Install pyenv (macOS/Linux)2curl https://pyenv.run | bash34# Install pyenv-virtualenv plugin5git clone https://github.com/pyenv/pyenv-virtualenv.git $(pyenv root)/plugins/pyenv-virtualenv67# Install Python version8pyenv install 3.8.0910# Create environment11pyenv virtualenv 3.8.0 myenv1213# Activate environment14pyenv activate myenv1516# Install packages17pip install package_name1819# Deactivate environment20pyenv deactivate
5. Pipenv
Pipenv is like a personal assistant for your Python projects. It handles the tedious tasks, letting you focus on the code that matters.
Kenneth Reitz, creator of the requests library and former Python Packaging Authority member, advocates for simpler tools:
While comprehensive tools like Conda have their place, I've found that for many Python projects, simpler is often better. That's why I created Pipenv – to strike a balance between the simplicity of pip and the reproducibility of virtual environments. The goal is to make dependency management in Python as painless as possible, especially for newcomers to the ecosystem.
Overview:
Combines pip and virtualenv for dependency and environment management
Pros:
Simplifies dependency management with Pipfile
Automates environment creation and package installation
Cons:
Can be slower than pip and venv separately
May struggle with complex dependency resolution
Usage:
1# Install pipenv2pip install pipenv34# Create environment and install dependencies5pipenv install67# Activate environment8pipenv shell910# Install specific package11pipenv install requests1213# Install development dependencies14pipenv install pytest --dev1516# Generate requirements.txt17pipenv lock -r > requirements.txt1819# Deactivate environment20exit
6. Poetry
Poetry brings harmony to the chaos of dependency management. It's like having a skilled conductor for your Python orchestra.
Overview:
Modern dependency management and packaging tool for Python
Pros:
Intuitive dependency resolution
Generates lock files for deterministic builds
Integrated build and publish functionality
Cons:
Steeper learning curve for beginners
May conflict with other tools in complex setups
Usage:
1# Install Poetry2curl -sSL https://install.python-poetry.org | python3 -34# Create a new project5poetry new myproject6cd myproject78# Add dependencies9poetry add requests1011# Add development dependency12poetry add pytest --group dev1314# Run commands in the virtual environment15poetry run python script.py1617# Generate requirements.txt18poetry export -f requirements.txt --output requirements.txt1920# Activate shell21poetry shell
7. Docker
Docker is the ultimate sandbox. It doesn't just isolate your Python environment; it isolates your entire development universe.
Overview:
Containerization platform that can be used for isolating Python environments
Pros:
Complete isolation of the entire development environment
Ensures consistency across different machines and operating systems
Can include non-Python dependencies and services
Cons:
Steeper learning curve
Requires more system resources
Complex to manage and understand
Usage:
1# Create a Dockerfile2cat << EOF > Dockerfile3FROM python:3.84WORKDIR /app5COPY requirements.txt .6RUN pip install -r requirements.txt7COPY . .8CMD ["python", "app.py"]9EOF1011# Build Docker image12docker build -t myproject .1314# Run container15docker run -it myproject1617# Run container with volume mount18docker run -it -v $(pwd):/app myproject
8. Hatch
Overview:
Modern, extensible Python project management tool
Pros:
Simplifies project creation and management
Supports multiple build backends
Includes built-in version management
Cons:
Relatively new, smaller community compared to other tools
Usage:
1# Install Hatch2pip install hatch34# Create a new project5hatch new myproject6cd myproject78# Run commands in the virtual environment9hatch run python script.py1011# Add dependency12hatch add requests1314# Run tests15hatch run test1617# Build project18hatch build
9. Manage Your Environments with Daytona
But why mess up your computer with all these different environment managers when you can use Daytona? Daytona offers a revolutionary approach to managing development environments, now with added GPU support:
Containerized Environments: Keep your laptop clean by using clearly separated and containerized dev environments.
Portability: Move your environments with you or host them on a remote server.
Consistency: Ensure all team members work in identical environments, eliminating "it works on my machine" issues.
Easy Setup: Create a fully configured development environment with a single command.
IDE Integration: Seamlessly works with popular IDEs like VS Code and JetBrains products.
Git Provider Integration: Easily connect with GitHub, GitLab, Bitbucket, and more for smooth workflow integration.
GPU Support: Leverage GPU acceleration directly within your Daytona workspaces, ideal for machine learning and data science projects.
With Daytona, you can forget about the complexities of managing Python environments on your local machine. Instead, you get a clean, portable, and consistent development environment that you can access from anywhere, complete with GPU capabilities when needed. Say goodbye to environment conflicts and hello to streamlined, efficient development with Daytona.
To get started with Daytona, simply install it and run:
1daytona create --code
This command will set up a new development environment and open it in your preferred IDE, ready for you to start coding immediately. No more worrying about Python versions, dependencies, or conflicting packages – Daytona handles it all for you in isolated, reproducible environments.
Leveraging Dev Container with Daytona
Daytona's support for devcontainer.json configuration files is one of its most powerful features, allowing you to define project-specific development environments with precision and ease. By including a devcontainer.json file in your project repository, you can specify not just the Python version and dependencies, but also any required system packages, VS Code extensions, environment variables, and even custom scripts to run during setup.
1{2 "name": "Python 3.8 Project",3 "image": "python:3.8",4 "extensions": [5 "ms-python.python",6 "ms-python.vscode-pylance"7 ],8 "settings": {9 "python.linting.pylintEnabled": true,10 "python.formatting.provider": "black"11 },12 "postCreateCommand": "pip install -r requirements.txt",13 "remoteUser": "vscode"14}
This means that every time you or a team member spins up a Daytona environment for your project, it will be configured exactly as needed, with all the tools and extensions required for optimal productivity. Whether you need specific linters, formatters, testing frameworks, or specialized tools for your project, they can all be automatically installed and configured.
This approach ensures consistency across your team, simplifies onboarding for new developers, and eliminates the "it works on my machine" problem once and for all. With Daytona and devcontainer.json, you're not just managing Python environments – you're creating complete, reproducible development environments tailored to each project's unique requirements. This powerful combination allows you to focus on coding and collaboration, knowing that your development environment is perfectly set up every time.
Best Practices for Python Environment Management
Version Pinning: Always specify exact versions of your dependencies in your requirements files or configuration. This ensures reproducibility across different setups. Use
==
for exact versions, e.g.,requests==2.26.0
.Use .gitignore: Add your virtual environment directories to your .gitignore file to prevent committing them to version control. A typical entry might look like:
1venv/2*.pyc3__pycache__/
Regular Updates: Periodically update your dependencies to benefit from bug fixes and security patches. Tools like
pip-compile
from pip-tools can help manage this process.Separate Production and Development Dependencies: Use separate requirements files or configuration sections for production and development dependencies. This keeps your production environment lean.
Use a consistent naming convention: Name your virtual environments consistently, perhaps based on the project name or functionality.
Document Your Setup: Include a README with clear instructions on how to set up the development environment for your project.
Automate Environment Setup: Use scripts or Makefiles to automate the creation and population of your development environments.
Use Environment Variables: Store sensitive information and configuration that varies between environments (like API keys) in environment variables rather than in your code.
Regularly Clean Up: Periodically remove unused virtual environments and dependencies to save disk space and reduce clutter.
Test in Clean Environments: Regularly test your project setup in a clean environment to ensure all dependencies are properly specified.
Conclusion
Choosing the right environment management tool depends on your specific needs, project requirements, and personal preferences. As one developer aptly stated:
The best tool is the one that solves your problems without creating new ones.
For beginners, starting with venv or virtualenv provides a solid foundation. As you grow more comfortable and your projects become more complex, you might find tools like Poetry or Conda more suitable. For those working in teams or on larger projects, solutions like Daytona offer comprehensive environment management with added benefits of consistency and portability.
Remember, the goal is to create a workflow that enhances your productivity and ensures your projects are easily reproducible. Experiment with different tools to find what works best for you, and don't hesitate to adapt your approach as your needs evolve.
Picking the right environment management tool is like choosing the right pair of shoes. What works for a marathon runner might not suit a mountain climber. Know your terrain and choose accordingly.