Have you ever wondered how to run AI-generated code in production safely? As AI agents become more sophisticated at generating code, the challenge isn't just about creating code—it's about executing it safely. To solve this critical challenge, this guide combines LangChain's powerful AI capabilities with Daytona's secure sandbox environments.
Picture LangChain as your AI code chef, cooking up sophisticated solutions, while Daytona acts as your secure test kitchen where these creations can be safely tried and tested. Together, they provide a robust framework for generating and executing AI code without compromising security.
TL;DR
Generate code confidently using LangChain's AI capabilities
Execute it securely in Daytona's isolated environments
Manage resources effectively at scale
Maintain complete control over the execution environment
Whether you're building AI-powered developer tools or implementing automated code generation in your pipeline, this integration provides the security and flexibility you need.
What is Daytona?
Daytona is an open-source development environment manager designed to create instant, fully-configured development environments across both local and cloud infrastructures. Its architecture supports deployment on all major cloud providers and platforms, while offering a self-hosted option ideal for enterprise and security-sensitive applications.
What sets Daytona apart is its comprehensive API for automated sandbox management through "Workspaces" - isolated development environments that provide secure boundaries for code execution. The platform offers native SDK support for both Python and TypeScript, making it seamless to integrate Daytona's capabilities into existing projects. With its robust infrastructure, Daytona enables automated environment provisioning, resource management, and secure code execution at scale.
Why Sandbox AI-Generated Code?
When working with AI-generated code, security is paramount. AI models might generate code that could:
Access system resources inappropriately
Make unauthorized network calls
Consume excessive memory or CPU resources
Perform changes to the system configuration
Daytona's workspace isolation provides a secure boundary for executing such code, making it an ideal solution for AI agent implementations.
What is LangChain?
LangChain is a framework designed to simplify the creation of applications using large language models (LLMs). It provides a modular architecture for combining LLMs with other tools and data sources, making it easier to build complex AI applications. The framework excels at tasks like prompt management, LLM output handling, and creating sophisticated chains of operations. For our use case, LangChain's ability to manage structured interactions with language models makes it an ideal choice for generating and processing code.
Building an AI Code Generator with LangChain and Daytona
Let's walk through creating a practical example that combines LangChain's code generation capabilities with Daytona's secure execution environment.
Setting Up the Environment
First, install the required packages:
1pip install langchain langchain-community openai daytona_sdk
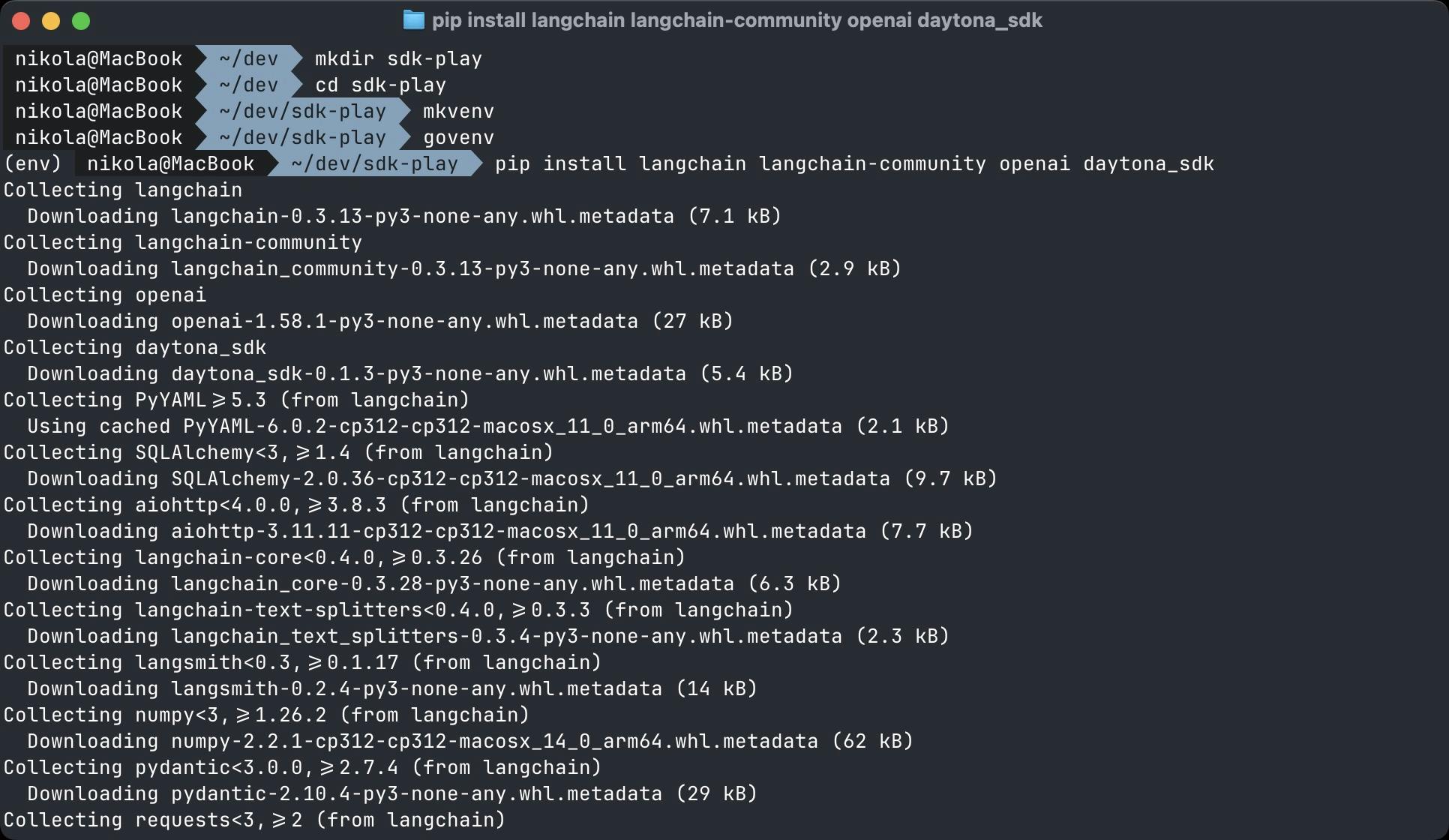
The Code Generation Component
Here's our implementation of a code generator using LangChain:
1from langchain_community.llms import OpenAI2from langchain.chains import LLMChain3from langchain.prompts import PromptTemplate4from langchain.chains import SimpleSequentialChain5import os67# Set your OpenAI API key8os.environ["OPENAI_API_KEY"] = "your-api-key-here"910# Create a prompt template for code generation11code_generation_prompt = PromptTemplate(12 input_variables=["language", "task"],13 template="""14You are an expert {language} developer. Write clean, efficient, and well-documented code for the following task:1516Task: {task}1718Please provide:191. A complete implementation202. Brief comments explaining key parts213. Example usage if applicable2223Code:24"""25)2627# Initialize the LLM with lower temperature for more focused code generation28llm = OpenAI(29 temperature=0.2,30 max_tokens=100031)3233# Create the code generation chain34code_chain = LLMChain(35 llm=llm,36 prompt=code_generation_prompt,37 verbose=True38)3940def generate_code(language: str, task: str):41 """42 Generate code for a given task in the specified programming language.43 """44 return code_chain.run({45 "language": language,46 "task": task47 })
Adding Daytona Integration
To safely execute the generated code, we'll now integrate Daytona into our workflow. The first step is to locate your Daytona API key and server URL. You can easily retrieve this information by running the command 'daytona config -k' in your terminal. This command will display both the URL and API key you need.
1from daytona_sdk import Daytona, DaytonaConfig, CreateWorkspaceParams23def execute_in_sandbox(code: str):4 """5 Execute generated code in a Daytona workspace sandbox.6 """7 # Initialize Daytona with your configuration8 config = DaytonaConfig(9 api_key="DAYTONA_API_KEY",10 server_url="DAYTONA_SERVER_URL",11 target="local"12 )13 daytona = Daytona(config=config)1415 # Create a Python workspace16 params = CreateWorkspaceParams(language="python")17 workspace = daytona.create()1819 try:20 # Execute the code in the sandbox21 response = workspace.process.code_run(code)2223 if response.code != 0:24 return f"Error: {response.code} {response.result}"25 return response.result26 finally:27 # Always clean up the workspace28 daytona.remove(workspace)2930# Example usage31if __name__ == "__main__":32 # Generate Python code33 task = "Create a function that takes a list of numbers and returns the moving average with a specified window size"34 generated_code = generate_code("Python", task)3536 # Execute in sandbox37 result = execute_in_sandbox(generated_code)38 print("Code execution result:", result)

Benefits of This Approach
Security: All code execution happens in isolated Daytona workspaces, preventing any potential system access or resource abuse.
Resource Management: Daytona workspaces can be configured with specific resource limits, ensuring controlled execution.
Clean Environment: Each execution starts in a fresh workspace, eliminating any potential side effects from previous runs.
Automated Cleanup: The workspace is automatically removed after execution, maintaining system cleanliness.
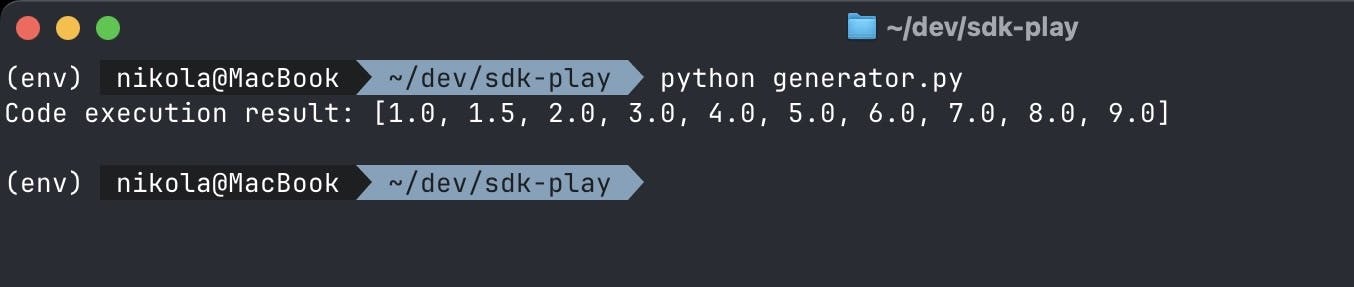
Built-in Features for Best Practices
Daytona simplifies the implementation of essential security and operational practices through its built-in features:
Resource Management and Scaling: Daytona's native infrastructure handles horizontal scaling across cloud resources automatically. Its simple configuration interface lets you set CPU, memory, and storage limits with just a few parameters, making resource management effortless.
Logging and Audit Controls: Built-in comprehensive logging captures all workspace activities automatically. Daytona provides ready-to-use APIs for tracking execution flows, resource usage patterns, and system interactions, giving you immediate visibility into your AI agent activities.
API Integration and Automation: Daytona comes with a full suite of APIs for file operations, git workflows, process management, and code analysis. These pre-built integrations make it straightforward to automate workflows and connect with existing systems without additional development effort.
Cost Control and Resource Optimization: Managing costs is simplified through Daytona's built-in spend controls and resource monitoring capabilities. The platform automatically tracks resource usage and provides easy-to-implement limits to optimize performance while controlling costs.
Security and Isolation: Security comes standard with Daytona's workspace isolation system. The platform automatically handles clean environment states, process timeouts, and security boundaries, ensuring safe code execution without manual configuration.
Get Started with Daytona SDK
Download our Python and TypeScript SDKs to manage development environments programmatically. Integrate Daytona's powerful features into your applications.
Conclusion
Combining AI code generation with Daytona's sandboxed execution creates a powerful and secure system for automated code generation and testing. This approach provides the flexibility to experiment with AI-generated code while maintaining robust security boundaries.
The solution we've built demonstrates how modern development tools can work together to create secure, efficient systems for AI-assisted development. Whether you're building an AI coding assistant or implementing automated code generation in your pipeline, Daytona provides the secure foundation needed for safe code execution.
Remember to replace the placeholder API keys with your actual Daytona and OpenAI credentials when implementing this solution in your environment.
What's Next?
In Part 2 of this series, we'll explore more advanced integration features of Daytona, focusing on using the filesystem API to manipulate files within the sandbox environment. We'll demonstrate how to:
Create and modify files within the workspace
Handle file uploads and downloads
Search for files and replace in files
Change file permissions and ownership
Stay tuned for more advanced implementations and best practices for working with Daytona's file manipulation capabilities.